通过使用Spring Boot+Spring MVC + Mybatis 整合实现一个对数据库中的users表的CRUD的操作。
1、创建项目
1.1、修改POM文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.2.6.RELEASE</version> <relativePath/> </parent> <groupId>com.xiezhenyu</groupId> <artifactId>springbootmybatis2</artifactId> <version>0.0.1-SNAPSHOT</version> <name>springbootmybatis2</name> <description>Demo project for Spring Boot</description> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.1.2</version> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid</artifactId> <version>1.1.12</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>8.0.18</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> </dependencies>
<build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> <resources> <resource> <directory>src/main/java</directory> <includes> <include>**/*.xml</include> <include>**/*.properties</include> </includes> <filtering>true</filtering> </resource> </resources> </build> </project>
|
1.2、添加application.properties全部配置文件
1 2 3 4 5 6
| spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver spring.datasource.url=jdbc:mysql://localhost:3306/test?userUnicode=true&characterEncoding=UTF-8&userSSL=false&serverTimezone=Asia/Shanghai spring.datasource.username=root spring.datasource.password=p123456 spring.datasource.type=com.alibaba.druid.pool.DruidDataSource mybatis.type-aliases-package=com.xiezhenyu.springbootmybatis2.pojo
|
1.3、数据库表设计
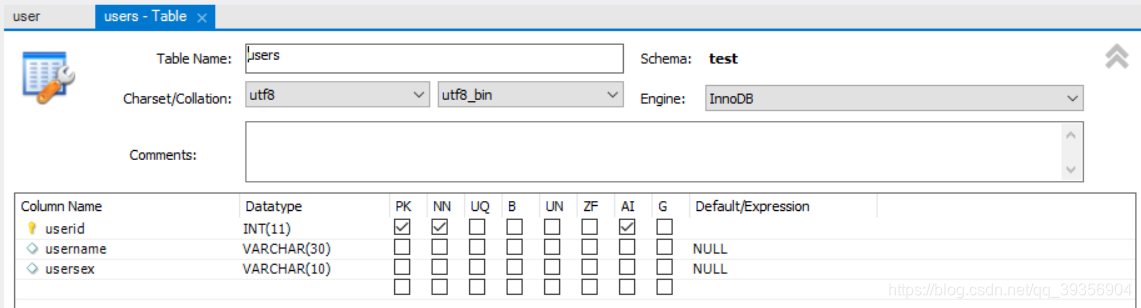
2、添加用户
2.1、创建实体类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| package com.xiezhenyu.springbootmybatis2.pojo;
public class Users { private Integer userid; private String username; private String usersex; public Integer getUserid() { return userid; } public void setUserid(Integer userid) { this.userid = userid; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public String getUsersex() { return usersex; } public void setUsersex(String usersex) { this.usersex = usersex; } }
|
2.2、创建mapper接口以及映射配置文件
mapper接口
1 2 3
| public interface UsersMapper { void insertUser(Users users); }
|
映射配置文件
1 2 3 4 5 6 7 8 9
| <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.xiezhenyu.springbootmybatis2.mapper.UsersMapper"> <insert id="inserUser" parameterType="users"> insert into users(username,usersex) values(#{username},#{usersex}) </insert> </mapper>
|
2.3、创建业务层
1 2 3 4 5 6 7 8 9
| public class UsersServiceImpl implements UsersService { @Autowired private UsersMapper usersMapper;
@Override public void addUser(Users users) { this.usersMapper.insertUser(users); } }
|
2.4、创建Controller
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| @Controller @RequestMapping("/users") public class UsersController { @Autowired private UsersService usersService;
@RequestMapping("/{page}") public String showPage(@PathVariable String page){ return page; }
@PostMapping("/addUser") public String addUser(Users users){ this.addUser(users); return "ok"; } }
|
2.5、编写页面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html xmlns:th="http://www.thymeleaf.org"> <head> <link rel="shortcut icon" href="../resource/favicon.ico" th:href="@{/static/favicon.ico}"/> <title>Title</title> </head> <body> <form th:action="@{/users/addUser}" method="post"> <input type="text" name="username"/><br/> <input type="text" name="usersex"/><br/> <input type="submit" value="ok"/> </form> </body> </html>
|
1 2 3 4 5 6 7 8 9 10
| <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Title</title> </head> <body> <span>操作成功!</span> </body> </html>
|
2.6、启动类
1 2 3 4 5 6 7
| @SpringBootApplication @MapperScan("com.xiezhenyu.mapper") public class Springbootmybatis2Application { public static void main(String[] args) { SpringApplication.run(Spirngbootmybatis2Application.class, args); } }
|
3、查询用户
3.1、创建mapper及映射配置文件
1 2 3 4
| public interface UsersMapper { void insertUser(Users users); List<Users> selectUsersAll(); }
|
1 2 3
| <select id="selectUsersAll" resultType="users"> select * from users </select>
|
3.2、在业务层中添加查询方法
1 2 3 4
| @Override public List<Users> findUserAll() { return this.usersMapper.selectUsersAll(); }
|
3.3、在Controller中添加方法
1 2 3 4 5 6 7 8 9
|
@RequestMapping("/findUserAll") public String findUser(Model model){ List<Users> list = this.usersService.findUserAll(); model.addAttribute("list",list); return "showUser"; }
|
3.4、编写页面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>Title</title> </head> <body> <table border="1" width="50%" align="center"> <tr> <th>ID</th> <th>用户名</th> <th>性别</th> <th>操作</th> </tr> <tr th:each="u : ${list}"> <td th:text="${u.userid}"></td> <td th:text="${u.username}"></td> <td th:text="${u.usersex}"></td> <td> <a th:href="@{/user/preUpdateUser(id=${u.userid})}">修改</a> <a th:href="@{/user/deleteUser(id=${u.userid})}">删除</a> </td> </tr> </table> </body> </html>
|
4、用户预更新
4.1、创建mapper及映射配置文件
1 2 3 4 5
| public interface UsersMapper { void insertUser(Users users); List<Users> selectUsersAll(); Users selectUserById(Integer id); }
|
1 2 3
| <select id="selectUserById" parameterType="Integer" resultType="users"> select * from users where userid = #{userid} </select>
|
4.2、在业务层中添加查询方法
1 2 3 4
| @Override public Users findUserById(Integer id) { return this.usersMapper.selectUserById(id); }
|
4.3、在Controller中添加方法
1 2 3 4 5 6 7 8 9
|
@RequestMapping("/preUpdateUser") public String findUserById(Integer id,Model model){ Users user = this.usersService.findUserById(id); model.addAttribute("user",user); return "updateUser"; }
|
4.4、编写页面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>Title</title> </head> <body> <form th:action="@{/user/updateUser}" method="post"> <input type="hidden" name="userid" th:value="${user.userid}"/> <input type="text" name="username" th:value="${user.username}"/><br/> <input type="text" name="usersex" th:value="${user.usersex}"/><br/> <input type="submit" value="修改"/> </form> </body> </html>
|
5、用户修改
5.1、创建mapper及映射配置文件
1 2 3 4 5 6
| public interface UsersMapper { void insertUser(Users users); List<Users> selectUsersAll(); Users selectUserById(Integer id); void updateUser(Users users); }
|
1 2 3
| <update id="updateUser" parameterType="users"> update users set username=#{username},usersex=#{usersex} where userid = #{userid} </update>
|
5.2、在业务层中添加查询方法
1 2 3 4
| @Override public void updateUser(Users users) { this.usersMapper.updateUser(users); }
|
5.3、在Controller中添加方法
1 2 3 4 5 6 7 8
|
@RequestMapping("updateUser") public String updateUser(Users users){ this.usersService.updateUser(users); return "ok"; }
|
6、用户删除
6.1、创建mapper及映射配置文件
1 2 3 4 5 6 7
| public interface UsersMapper { void insertUser(Users users); List<Users> selectUsersAll(); Users selectUserById(Integer id); void updateUser(Users users); void deleteUserById(Integer id); }
|
1 2 3
| <delete id="deleteUserById" parameterType="Integer"> delete from users where userid = #{userid} </delete>
|
6.2、在业务层中添加查询方法
1 2 3 4
| @Override public void deleteUserById(Integer id) { this.usersMapper.deleteUserById(id); }
|
6.3、在Controller中添加方法
1 2 3 4 5 6 7 8
|
@RequestMapping("deleteUser") public String deleteUserById(Integer id){ this.usersService.deleteUserById(id); return "ok"; }
|